Personal Growth
Building a Navigation Component with Variables
Feb 6, 2022
Introduction
Navigation components are essential elements of any web or mobile application, providing users with a way to move through different sections of a site or app. Building a navigation component using variables can enhance its flexibility and maintainability. Here’s how to create a dynamic navigation component with variables.
Understanding Variables in Navigation Components
What Are Variables?
In the context of web development, variables are containers that store data values. Using variables in navigation components allows you to dynamically change properties like labels, links, and styles without modifying the underlying code structure. This makes your navigation component more adaptable and easier to update.
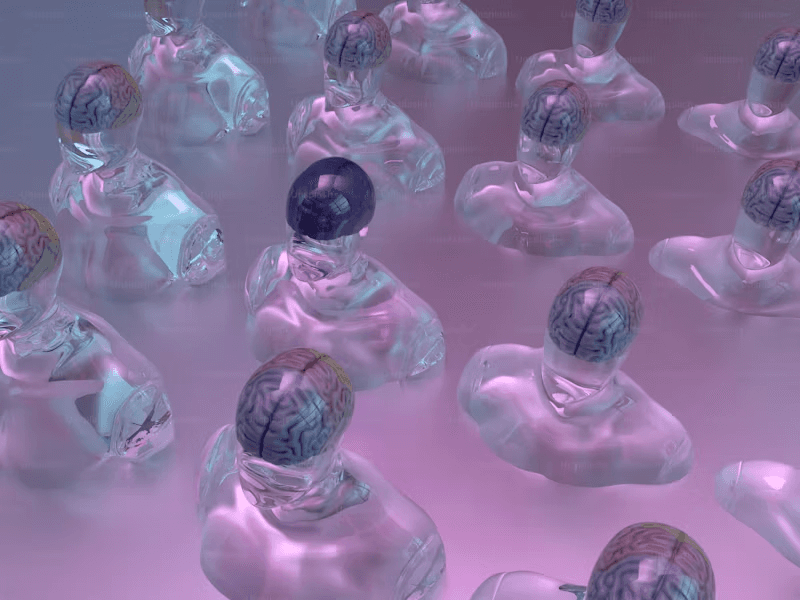
Benefits of Using Variables
Using variables in navigation components offers several benefits:
Flexibility: Easily update navigation links and labels.
Maintainability: Simplify updates and reduce the risk of errors.
Consistency: Ensure uniformity across different parts of your application.
Steps to Build a Navigation Component with Variables
Step 1: Define Variables
Start by defining the variables you’ll use in your navigation component. This can include variables for link names, URLs, and any other dynamic properties. For example:
const navLinks = [ { name: 'Home', url: '/' }, { name: 'About', url: '/about' }, { name: 'Services', url: '/services' }, { name: 'Contact', url: '/contact' }, ];
Step 2: Create the Navigation Structure
Next, create the HTML or JSX structure for your navigation component. Use a loop to dynamically generate navigation links based on the variables defined:
const Navigation = () => { return ( <nav> <ul> {navLinks.map((link, index) => ( <li key={index}> <a href={link.url}>{link.name}</a> </li> ))} </ul> </nav> ); };
Step 3: Style the Navigation Component
Style your navigation component using CSS variables to ensure consistency and easy updates. Define your CSS variables in a root block:
:root { --nav-bg-color: #333; --nav-text-color: #fff; } nav { background-color: var(--nav-bg-color); color: var(--nav-text-color); }
Step 4: Implement Dynamic Behavior
Enhance your navigation component with dynamic behavior using JavaScript or a JavaScript framework. For example, you can highlight the active link based on the current URL:
const Navigation = () => { const currentPath = window.location.pathname; return ( <nav> <ul> {navLinks.map((link, index) => ( <li key={index} className={currentPath === link.url ? 'active' : ''}> <a href={link.url}>{link.name}</a> </li> ))} </ul> </nav> ); };
Conclusion
Building a navigation component with variables enhances its flexibility, maintainability, and consistency. By defining variables for links, URLs, and styles, you can create a dynamic and adaptable navigation system that is easy to update and manage. This approach not only improves the user experience but also simplifies the development and maintenance process.
Related Articles
CALL TO ACTION SECTION
Are you ready to be productive?
Join over +100.000 users and teams in the community
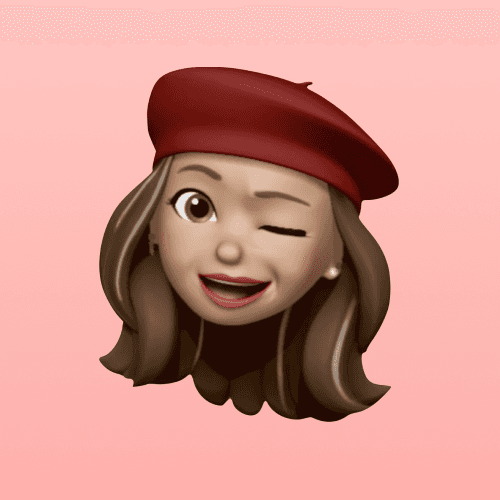




